The Eleventh Day of Tiny Code
Tiny Code Christmas - Day 11⌗
Welcome to Day 11 of Tiny Code Christmas! If you’re just joining us don’t forget to check out the overview, and start at Day 1! You can start whenever you like, there are no deadlines!
Today we are going to enter the third dimension and build a voxel cube! Optionally, there is sample code under the challenge section to accompany this that you can use.
If you’re following along on PICO-8, you will need the full version to meet some of the size challenges today.
The Challenge: The Third Dimension⌗
The first challenge is to create a voxel cube that rotates around two axes, the voxel cube must have an additional effect incorporated. This can be an effect like coloring the voxels or it can be a movement effect.
You can check out our Day 11 video above for an overview on this structure, the code mentioned in the video is below.
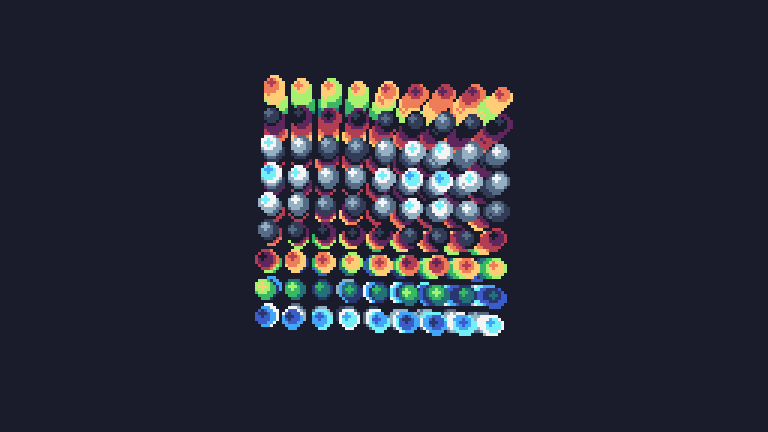
The second challenge is to keep your effect at a maximum of 256 BYTES! We are on to the compressed file formats now so lets see how many extra bytes we can squeeze in!
The Expert Challenge⌗
These expert challenges will push your sizecoding knowledge to the limit! Don’t forget to check out our previous Expert Challenges!
- A hollow cube
- 256 characters
- No line or triangle functions
Sample Code for TIC-80⌗
sin=math.sin
cos=math.cos
angle=0
function rotatey(p,angle)
xt = p.x*cos(angle) - p.z*sin(angle)
yt = p.y
zt = p.x*sin(angle) + p.z*cos(angle)
return {x=xt,y=yt,z=zt}
end
function rotatex(p,angle)
xt = p.x
yt = p.y*cos(angle) - p.z*sin(angle)
zt = p.y*sin(angle) + p.z*cos(angle)
return {x=xt,y=yt,z=zt}
end
function rotatez(p,angle)
xt = p.x*cos(angle) - p.y*sin(angle)
yt = p.x*sin(angle) + p.y*cos(angle)
zt = p.z
return {x=xt,y=yt,z=zt}
end
function TIC()
cls()
t=time()/200
points = {}
angle=angle+.01
for x=-25,25,6 do
for y=-25,25,6 do
for z=-25,25,6 do
p=rotatez({x=x,y=y,z=z},angle)
q=rotatex(p,angle)
r=rotatey(q,angle)
table.insert(points,
{x=r.x,y=r.y,z=r.z+400})
end
end
end
table.sort(points,
function (a,b) return a.z>b.z end
)
for i=1,#points do
for j=0,2 do
circ(120+600*points[i].x/points[i].z-j/2,
68+600*points[i].y/points[i].z-j/2,
3-j,
14-j);
end
end
end
Sample Code for PICO-8⌗
angle=0
function rotatey(p,angle)
xt = p.x*cos(angle) - p.z*sin(angle)
yt = p.y
zt = p.x*sin(angle) + p.z*cos(angle)
return {x=xt,y=yt,z=zt}
end
function rotatex(p,angle)
xt = p.x
yt = p.y*cos(angle) - p.z*sin(angle)
zt = p.y*sin(angle) + p.z*cos(angle)
return {x=xt,y=yt,z=zt}
end
function rotatez(p,angle)
xt = p.x*cos(angle) - p.y*sin(angle)
yt = p.x*sin(angle) + p.y*cos(angle)
zt = p.z
return {x=xt,y=yt,z=zt}
end
function zsort(points)
for i=1,#points do
local j = i
while j > 1 and points[j-1].z<points[j].z do
points[j-1],points[j] = points[j],points[j-1]
j = j-1
end
end
end
function _draw()
cls()
t=time()
points = {}
angle=angle+.01
for x=-12,12,6 do
for y=-12,12,6 do
for z=-12,12,6 do
p=rotatez({x=x,y=y,z=z},angle)
q=rotatex(p,angle)
r=rotatey(q,angle)
add(points,
{x=r.x,y=r.y,z=r.z+400})
end
end
end
zsort(points)
for i=1,#points do
for j=0,2 do
circfill(64+600*points[i].x/points[i].z-j/2,
64+600*points[i].y/points[i].z-j/2,
3-j,
13-j);
end
end
end
Sharing is Caring!⌗
If you feel like it, why not share what you’ve done with us on the LoveByte Discord, #lovebyte on IRCnet, or share on Twitter and Mastodon using the hashtag #lovebytetcc